Advanced workshop
Architecting Angular Apps for High Performance
High-Speed Angular applications on any device
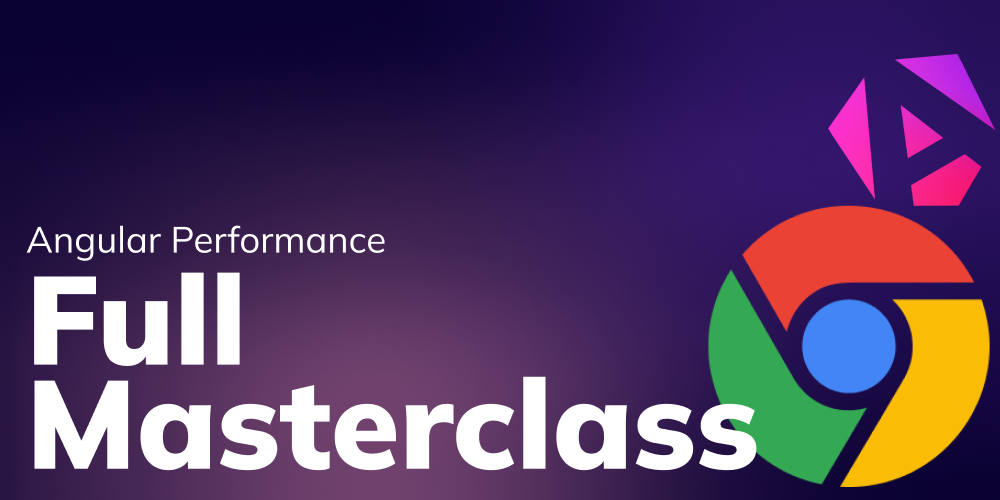
Advanced workshop
High-Speed Angular applications on any device
This is the second post in a three-part series unveiling the secret capabilities of Zone-Flags and the hidden pitfalls of disabling Zone, configuring it statically, or migrating it. In the previous article, we discussed how Angular's change detection mechanism worked, and the last part of this series will go into more detail on the Zone-Flags debugging and risks related to configuring Zone over Zone-Flags.
Zone-Flags prove helpful indeed when it comes to configuring Zone. You can use them to substantially improve the performance of your Angular app and better orchestrate the change detection process.
So, let's get a first glance at what Zone-Flags are, what opportunities they offer, and what to expect configuring them in your project.
⚠️ Notice
Zone-Flags are a static way to partially disable zone.js.
This means configuration has to happen statically before zone.js is imported. We will discuss later in the article what consequences doing otherwise might have.
Zone-Flags are Symbols on the window object, so we can use the direct window properties to set them up. Accordingly, upon initialization, Zone starts looking for specific flags in the window object to see if certain APIs should be patched or not.
Besides the global window object, zone-flags
To use Zone-Flags, first, you should create a file in your src folder named zone-flags.ts
When zone.js is initialized on the page, it takes on the values of the flags that are already located in the window
zone-flags.ts
zone.js
polyfills.ts
zone-flags.ts
Then, you can easily test it and see what happens if flags are not imported. Start your Angular application with the flags file commented out and run a measurement:
At this point, there are two more things that we need to keep in mind.
First, all flags’ configurations should be imported from a separate file (window as any
zone-flags.ts
zone-flags
zone.js
polyfills.ts
Second, files must be imported before any executable code. The reason behind it is that all imports normally get hoisted by webpack, and thus the imported code is injected into a bundle before any meaningful JS in the file. Here is an example of what happens if you neglect this rule.
The input:
The output produced by webpack compilation:
It is inefficient to do so, and I cannot emphasize enough how important it is to learn to do things the right way.
We already know from the previous article that it is preferable to configure zone.js instead of fully disabling it. This way, you can gain more control over your app – which is especially important when the application is big and complex – but this will only work out if the configuration is applied gradually. This is where Zone-Flags should come into action.
The default approach allows setting up Zone-Flags in three steps.
1. First, you need to create zone-flags.ts
polyfills.ts
2. Then, in your zone-flags.ts
3. Finally, import zone-flags.ts above the zone in polyfills.ts
This way of setting up zone-flags
With RxAngular helpers in place, it is easier and more convenient to set up zone-flags
Comprehensive and well-maintained documentation
Typed methods
Autocompletion
Inline documentation
Predefined event names
Convenience methods
Assertions for checking if zone-flags are correctly used
Keeping them in mind, let’s see how zone-flags
The first and last steps in the setup process will basically be the same. You begin your zone-flags
zone-flags.ts
polyfills.ts
zone-flags.ts
polyfills.ts
That said, the big change that makes all the difference here is the content you insert into your zone-flags.ts
In this file, we are disabling some global APIs and several DOM events by adding typed and extra convenience methods.
As you type, you will see zoneConfig
Every API has a speaking name chained with dot-notation to specify its type – e.g., Disable or Unpatched events:
As I previously pointed out, autocompletion is one of the perks you can expect when setting up zone-flags
After Zone-Flags are set up, their default runtime configuration can also be changed. First, you need to create a zone-runtime.ts
polyfills.ts
Then, open your polyfills.ts
⚠️ Notice
errors if it is used incorrectly. If you used zone-runtime configurations wrong (not executing it after zone.js runs) you should see the following error in the console: @rx-angular/cdk/zone-configuration
Now your zone-flags
As you’ve already seen, there are certain risks to be aware of when working with zone.js. Therefore, in the next article, we will find out how to mitigate them and effectively debug zone-flags