Angular 19.2: Template Literals in [class] – A Cleaner Alternative to [ngClass]?
Table of Contents
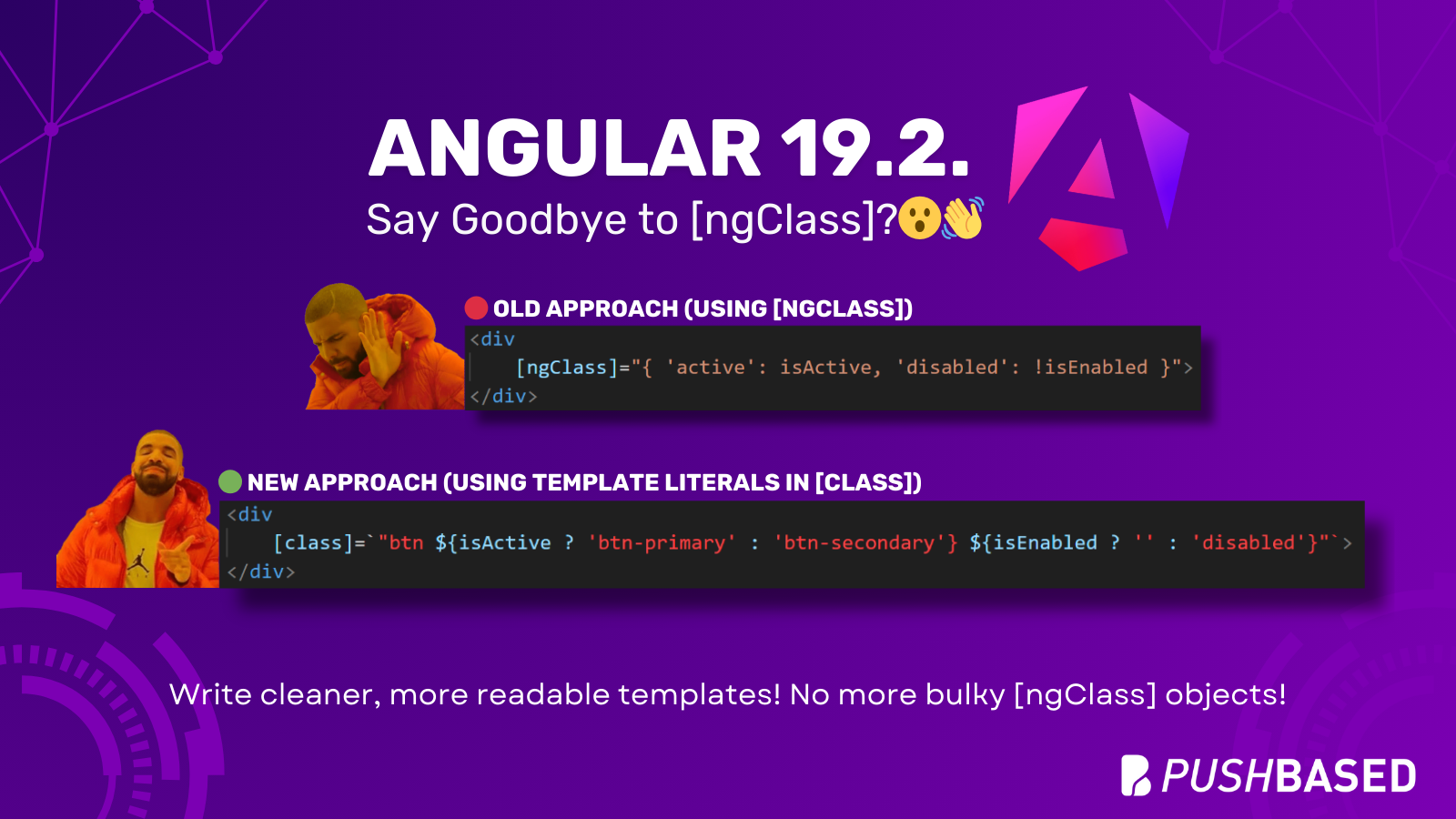
Angular 19.2 introduces a powerful enhancement for dynamic styling—template literals inside [class]
[ngClass]
[ngStyle]
Let’s explore what’s new, why it matters, and how to use it effectively.
The Problem: How We Used to Handle Dynamic Classes
Before Angular 19.2, dynamically assigning multiple classes required one of these approaches:
Using [ngClass]
for Conditional Styling
[ngClass]
<div [ngClass]="{ 'active': isActive, 'disabled': !isEnabled }"></div>
This approach works, but it can get cumbersome when dealing with multiple conditions.
Concatenating Classes Dynamically with Functions
<div [class]="getClasses()"></div>
Here, getClasses()
The Angular 19.2 Solution: Template Literals in [class]
Angular 19.2 allows direct template literals inside [class]
[ngClass]
How It Works
<div [class]=`"btn ${isActive ? 'btn-primary' : 'btn-secondary'} ${isEnabled ? '' : 'disabled'}"`></div>
Why This Is Better
Cleaner syntax
No need for an object structure or additional functions.
More readable templates
No extra brackets or function calls.
Native JavaScript syntax
Uses a familiar approach for developers.
A Real-World Example: User Profile Card
Imagine you’re building a user profile card that:
✅ Adapts to different themes (premium, standard).
✅ Reflects the user’s online/offline status.
✅ Dynamically applies the user’s favorite color.
Here’s how you can achieve this using template literals in [class]
[style]
What’s Happening Here?
Dynamic Classes:
orcard-premium
is applied based oncard-standard
user.isPremium
Online/Offline Status:
The
oronline
class changes dynamicallyoffline
Dynamic Inline Styles:
The background color and font size adjust according to user preferences.
This eliminates extra function calls while keeping the template concise and readable.
When to Use Template Literals vs. [ngClass]
While template literals in [class]
[ngClass]
Scenario | Use Template Literals | Use |
Few conditional classes | ✅ | ❌ |
Complex class conditions | ❌ | ✅ |
Performance-sensitive templates | ✅ | ❌ |
Multiple independent class bindings | ❌ | ✅ |
When [ngClass] Is Still Useful
When you need conditional logic for many classes
When binding objects or dynamically generating many class names
When your logic is too complex for inline expressions
Final Thoughts
Angular 19.2’s support for template literals in [class]
[ngClass]
Are you ready to switch?