Unlocking Code's DNA: What You Need to Know About Abstract Syntax Trees
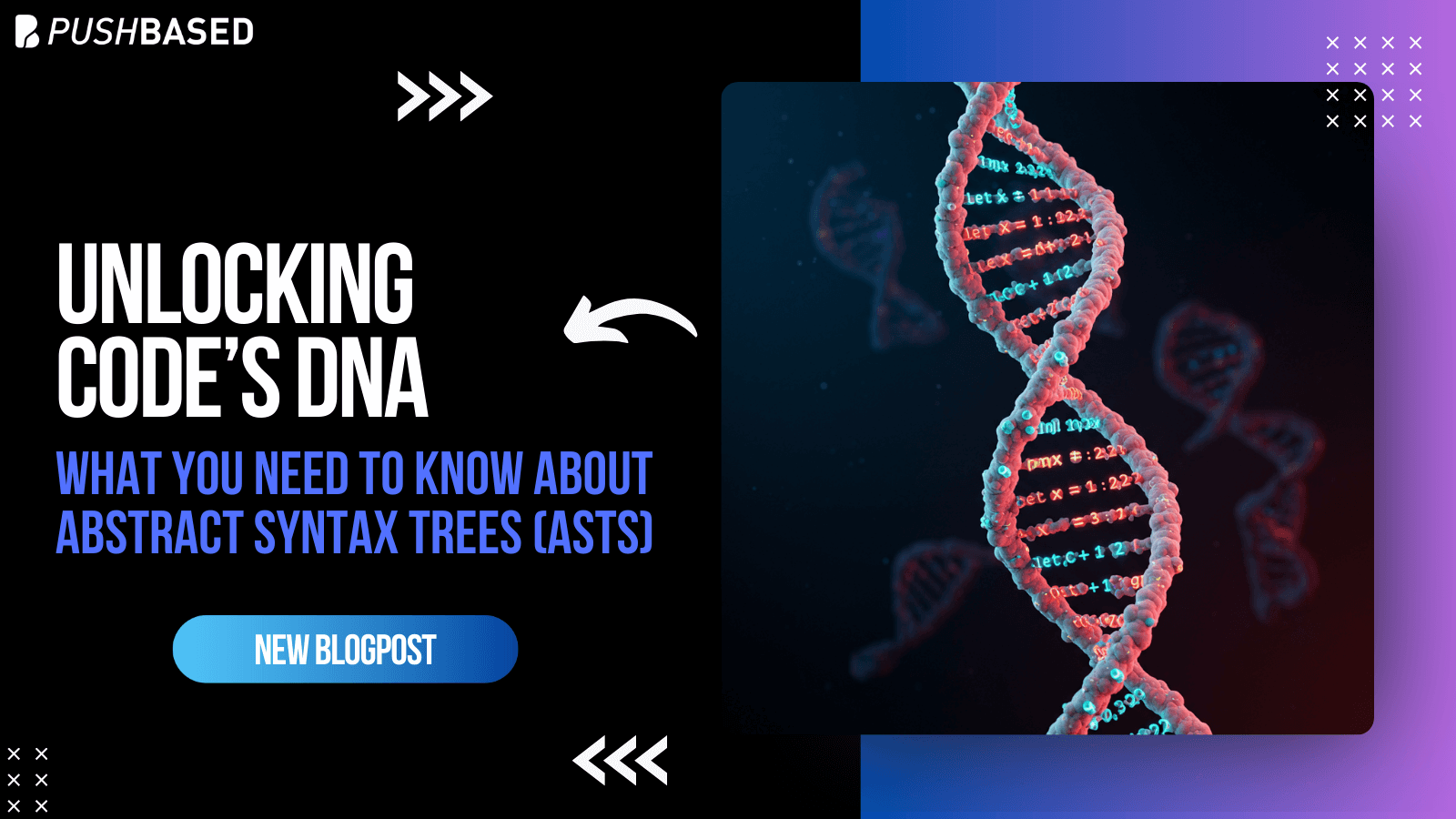
Ever peek under the hood of your favorite coding tools and wonder how they really work? It's not magic (though it sometimes feels like it!). It's all thanks to something called an Abstract Syntax Tree, or AST. Think of an AST as the secret blueprint, the hidden structure that reveals the true meaning of your code – way more than just the lines you see on the screen. Let's dive in and demystify these ASTs, and I promise you'll see your code (and your tools) in a whole new light.
From Text to Tree: What the Heck Is an AST Anyway?
Let's say you're building something awesome with LEGOs. You've got all these individual bricks – your keywords, your variables, your operators. But without instructions, it's just a pile of plastic. The AST is like those LEGO instructions, but for your code. It shows you how everything fits together to build something functional.
It's not the code itself. It's a representation of the code's structure – a way to organize it that computers can easily understand.
Check out this simple JavaScript:
let x = 1 + 2;
A simplified version of its AST might look like this (imagine it as a tree diagram):
See what's happening here?
It breaks things down:
We can see the variable declaration
, the assignment(let x)
, and the actual calculation(=)
all separated out.(1 + 2)
It shows how things connect: It's crystal clear that
and1
are being added together, and that result is being Assigned to2
.x
It ignores the fluff: Extra spaces, comments? The AST doesn't care! It's all about the meaning of the code, not how it looks.
That tree structure is the key! It lets computers (and the clever tools we build) actually reason about your code. It's no longer just a jumble of text – it's a well-organized set of instructions.
ASTs in Action: The Secret Sauce of Your Favorite Tools
Okay, cool theory, but how does this actually help you? Let's look at two everyday examples: ESLint and those life-saving code migrations.
1. ESLint: Your Code's Personal Trainer
ESLint (and other linters) are like personal trainers for your code – they keep it in shape and catch those little errors before they become big problems. But how do they know you made a mistake? You guessed it: ASTs!
Example: The no-unused-vars
Think about the no-unused-vars
Parsing: First, ESLint takes your code and transforms it into an AST.
Walking the Tree: Then, it carefully "walks" through that AST, visiting each part of the tree.
Detective Work: For every variable declaration, it makes a note of the variable's name. Then it looks around for any other parts of the tree that use that variable.
The Verdict: If, after checking the whole tree, a variable has only been declared but never used, ESLint throws up that helpful warning.
Without the AST, ESLint would be stuck trying to understand your code with messy string matching and regular expressions – a total recipe for disaster! The AST gives it a reliable, consistent way to analyze your code's structure.
2. Code Migrations: Upgrades Without the Headache
Ever had to update a huge project to a new version of a framework (React, Angular, Vue – you name it)? Breaking changes, renamed functions, different component structures... a total pain, right? Enter code migration tools, often called "codemods" – and they're powered by, you guessed it, ASTs!
Real-World Scenario: Changing a Function Name
Imagine a framework decides to rename a function from oldFunction
newFunction
Parse Everything: It reads your entire codebase and builds ASTs for every single file.
Find the Matches:
It goes through each AST, looking for any function calls (
nodes) where the function being called (CallExpression
) iscallee
.oldFunction
Make the Switch:
When it finds a match, it carefully modifies the AST, changing the function name to
.newFunction
Write it Back: Finally, it takes that updated AST and generates the new, corrected code.
This is incredibly precise and safe. Because the codemod is working with the AST, it understands the code's context. It won't accidentally rename a variable named oldFunction
But Wait, There's More!
ESLint and codemods are just the beginning. ASTs are used everywhere:
Babel: That magical tool that lets you use fancy new JavaScript features even in older browsers? Yep, ASTs.
Prettier: Automatically formats your code so it's nice and consistent? ASTs again.
Syntax Highlighters: How do those editors know what color to make your code? You get the idea.
Code Analysis Tools: Finding bugs, performance issues, security risks... all powered by ASTs.
Typescript: Uses ASTs to check and infer types.
Go Explore the AST! (Seriously!)
The best way to really "get" ASTs is to see them. Head over to AST Explorer. This awesome website lets you paste in code (JavaScript, CSS, HTML, and more) and instantly see the AST. Play around with different code snippets, different languages, and different parsers. It's like having X-ray vision for your code!
The Unsung Hero of Modern Development
ASTs might seem a bit abstract (pun intended!), but they're the foundation of so many tools we developers rely on every single day. By understanding ASTs, you're not just learning a cool technical concept – you're gaining a deeper understanding of how your code works and how to make it even better. So go explore, experiment, and unlock the power of the AST!
More Content incoming!
Visitor Pattern!
How to write an ESLint rule
How to write migration schematics
Stay tuned! 🚀